Write a device driver for Zephyr - Part 2
This is the second post in this series. See also part part1, part3 and part4.
Overview
In the first part1 of this series, we did setup the hardware and prepared the software environment. In this part we will focus on pretty much everything but writing the actual driver implementation. We will touch multiple areas in order to fully integrate the driver into the Zephyr project, this includes:
- Devicetrees
- The driver
- KConfig
- Unit tests
Lets introduce each one of those before we start.
Devicetrees
A Devicetree [2] is a data structure that describe the static hardware configuration in a standard manner. One of the motivations behind devicetree is that it should not be specific for any kernel. In the best of the worlds, you should be able to boot a Linux kernel, BSD kernel or Zephyr (well..) with the same devicetree. I've never heard about a working example IRL though, but the idea is good.
In the same way, you should be able to boot the same kernel on different board by only swap the devicetree. In Zephyr, the devicetree is integrated to the binary blob, so this idea does not fully apply to Zephyr though.
There are two types of files related to device trees in Zephyr:
- Devicetree sources - the devicetree itself (including dts, interface files and overlays).
- Devicetree bindings - description of its content. E.g. data types and which properties that is required or optional.
Zephyr does make use of both of these type of files during the build process. It allows the build process to make a build-time validation of the devicetree sources against the bindings, generate KConfig macros and a whole bunch of other macros that is to be used by the application and by Zephyr itself. We will see example of these macros later on.
Here is a simplified picture of the build process with respect to devicetrees:
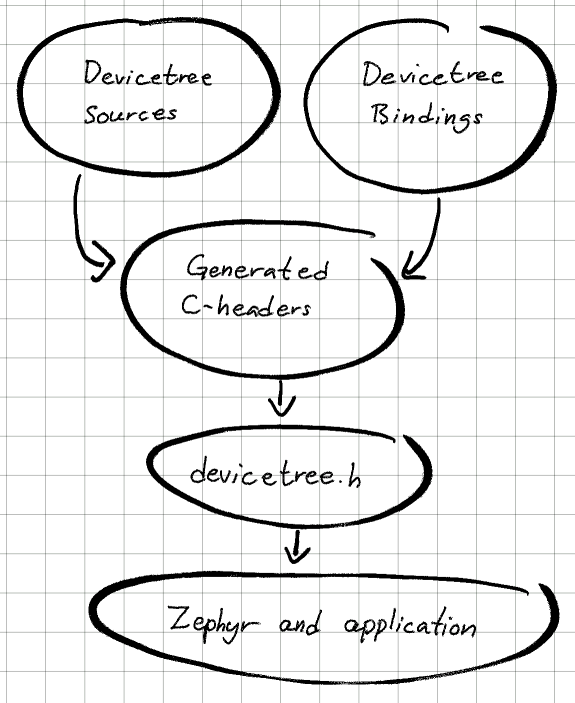
Driver
All drivers is located in the ./driver directory. It's C-files that contains the actual implementation of the driver.
KConfig
Like the Linux kernel (and U-boot, busybox, Barebox, Buildroot...), Zephyr uses the KConfig system to select what subsystem, libraries and drivers to be included in the build.
Remember when we did build the blinky application in the part1? We did provide -b rpi_pico to the build command to specify board:
west build -b rpi_pico ....
This will load ./boards/arm/rpi_pico/rpi_pico_defconfig as the default configuration and store it into ./build/zephyr/.config, which is the actual configuration the build system will use.
The .config file contains all configuration options selected by e.g. menuconfig AND the generated configuration options from the devicetree.
Unit tests
Zephyr makes use of Twister [1] for unit tests. By default it will build the majority of all tests on a defined set of boards. All these tests is part of the automatic test procedure for every pull request.
Lets start!
First we have to create a few files and integrate them into the build system. The directory hiearchy is similiar to the Linux kernel, lucky for me, it was quite obvious where to put things.
Driver
Create an empty file for now:
touch drivers/dac/dac_ltc166x.c
The driver will support both ltc1660 (10-bit, 8 channels) and ltc1665 (8-bit, 8 channels) DAC. I do not prefer to name drivers with an x as there actually are chips out there with an x in their name, so it could be a little fraudulent. That is at least something we try to avoid it in the Linux kernel.
A better name would be just dac_ltc1660.c and support all ICs that are compatible with dac_ltc1660. However, the Zephyr project has choosen to make use of the x in names to indicate that multiple chips are supported. When in Rome, do as the Romans do.
Add the file to the CMake build system:
diff --git a/drivers/dac/CMakeLists.txt b/drivers/dac/CMakeLists.txt index b0e86e3bd4..800bc895fd 100644 --- a/drivers/dac/CMakeLists.txt +++ b/drivers/dac/CMakeLists.txt @@ -9,6 +9,7 @@ zephyr_library_sources_ifdef(CONFIG_DAC_SAM dac_sam.c) zephyr_library_sources_ifdef(CONFIG_DAC_SAM0 dac_sam0.c) zephyr_library_sources_ifdef(CONFIG_DAC_DACX0508 dac_dacx0508.c) zephyr_library_sources_ifdef(CONFIG_DAC_DACX3608 dac_dacx3608.c) +zephyr_library_sources_ifdef(CONFIG_DAC_LTC166X dac_ltc166x.c) zephyr_library_sources_ifdef(CONFIG_DAC_SHELL dac_shell.c) zephyr_library_sources_ifdef(CONFIG_DAC_MCP4725 dac_mcp4725.c) zephyr_library_sources_ifdef(CONFIG_DAC_MCP4728 dac_mcp4728.c)
CONFIG_DAC_LTC166X comes from the Kconfig system and could be either 'y' or 'n' dependig on if it's selected or not.
Kconfig
Create two new Kconfig configuration options. One for the driver itself and one for its init priority:
diff --git a/drivers/dac/Kconfig.ltc166x b/drivers/dac/Kconfig.ltc166x new file mode 100644 index 0000000000..6053bc39bf --- /dev/null +++ b/drivers/dac/Kconfig.ltc166x @@ -0,0 +1,22 @@ +# DAC configuration options + +# Copyright (C) 2023 Marcus Folkesson <marcus.folkesson@gmail.com> +# +# SPDX-License-Identifier: Apache-2.0 + +config DAC_LTC166X + bool "Linear Technology LTC166X DAC" + default y + select SPI + depends on DT_HAS_LLTC_LTC1660_ENABLED || \ + DT_HAS_LLTC_LTC1665_ENABLED + help + Enable the driver for the Linear Technology LTC166X DAC + +if DAC_LTC166X + +config DAC_LTC166X_INIT_PRIORITY + int "Init priority" + default 80 + help + Linear Technology LTC166X DAC device driver initialization priority. + +endif # DAC_LTC166X
DT_HAS_LLTC_LTC1660_ENABLED and DT_HAS_LLTC_LTC1660_ENABLED is configuration options that is generated from the seleted devicetree. By depend on it, the DAC_LTC166X option will only show up if there are such a node specified. I really like this feature.
Also add it into the build stucture:
diff --git a/drivers/dac/Kconfig b/drivers/dac/Kconfig index 7b54572146..77b0db902b 100644 --- a/drivers/dac/Kconfig +++ b/drivers/dac/Kconfig @@ -42,6 +42,8 @@ source "drivers/dac/Kconfig.dacx0508" source "drivers/dac/Kconfig.dacx3608" +source "drivers/dac/Kconfig.ltc166x" + source "drivers/dac/Kconfig.mcp4725" source "drivers/dac/Kconfig.mcp4728"
Device tree
The bindings for all devices has to be described in the YAML format. These bindings is verified during compile time in order to make sure that the device tree node fulfills all required properties and not tries to invent some new ones. This protects us against typos, which also is a really good feature. The Linux kernel does not have this...
We have to create such a binding, one for each chip:
diff --git a/dts/bindings/dac/lltc,ltc1660.yaml b/dts/bindings/dac/lltc,ltc1660.yaml new file mode 100644 index 0000000000..196204236a --- /dev/null +++ b/dts/bindings/dac/lltc,ltc1660.yaml @@ -0,0 +1,8 @@ +# Copyright (C) 2023 Marcus Folkesson <marcus.folkesson@gmail.com> +# SPDX-License-Identifier: Apache-2.0 + +include: [dac-controller.yaml, spi-device.yaml] + +description: Linear Technology Micropower octal 10-Bit DAC + +compatible: "lltc,ltc1660" diff --git a/dts/bindings/dac/lltc,ltc1665.yaml b/dts/bindings/dac/lltc,ltc1665.yaml new file mode 100644 index 0000000000..2c789ecc56 --- /dev/null +++ b/dts/bindings/dac/lltc,ltc1665.yaml @@ -0,0 +1,8 @@ +# Copyright (C) 2023 Marcus Folkesson <marcus.folkesson@gmail.com> +# SPDX-License-Identifier: Apache-2.0 + +include: [dac-controller.yaml, spi-device.yaml] + +description: Linear Technology Micropower octal 8-Bit DAC + +compatible: "lltc,ltc1665"
dac-controller.yaml and spi-device.yaml is included to inherit some of the required properties (such as spi-max-speed) for this of device.
Unit tests
Add the driver to the test framework and allow the test to be executed on the native_posix platform:
diff --git a/tests/drivers/build_all/dac/testcase.yaml b/tests/drivers/build_all/dac/testcase.yaml index fa2eb5ac7a..1c7fa521d0 100644 --- a/tests/drivers/build_all/dac/testcase.yaml +++ b/tests/drivers/build_all/dac/testcase.yaml @@ -5,7 +5,7 @@ tests: drivers.dac.build: # will cover I2C, SPI based drivers platform_allow: native_posix - tags: dac_dacx0508 dac_dacx3608 dac_mcp4725 dac_mcp4728 + tags: dac_dacx0508 dac_dacx3608 dac_mcp4725 dac_mcp4728 dac_ltc1660 dac_ltc1665 extra_args: "CONFIG_GPIO=y" drivers.dac.mcux.build: platform_allow: frdm_k22f
Also add nodes in app.overlay to make it possible for the unit tests to instantiate the DAC:
diff --git a/tests/drivers/build_all/dac/app.overlay b/tests/drivers/build_all/dac/app.overlay index 471bfae6e8..c1e9146974 100644 --- a/tests/drivers/build_all/dac/app.overlay +++ b/tests/drivers/build_all/dac/app.overlay @@ -68,6 +68,8 @@ /* one entry for every devices at spi.dtsi */ cs-gpios = <&test_gpio 0 0>, + <&test_gpio 0 0>, + <&test_gpio 0 0>, <&test_gpio 0 0>, <&test_gpio 0 0>; @@ -118,6 +120,20 @@ channel6-gain = <0>; channel7-gain = <0>; }; + + test_spi_ltc1660: ltc1660@3 { + compatible = "lltc,ltc1660"; + reg = <0x3>; + spi-max-frequency = <0>; + #io-channel-cells = <1>; + }; + + test_spi_ltc1665: ltc1665@4 { + compatible = "lltc,ltc1665"; + reg = <0x4>; + spi-max-frequency = <0>; + #io-channel-cells = <1>; + }; }; }; };
Summary
It are some work that needs to be done to integrate the driver into the Zephyr project. This has to be done for every driver.
In part3 we will start writing the driver code.